mirror of
https://github.com/crypto-pro-web/crypto-pro-js.git
synced 2025-04-12 00:33:05 +03:00
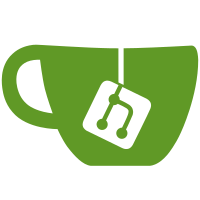
* Добавил новые методы для получения сертификатов * Методы загрузки объединённого списка сертификатов из личного хранилища и из закрытого ключа * Поиск сертификата в объединённом списке сертификатов из личного хранилища и из закрытого ключа * Получение сертификата в формате Cades из личного хранилища пользователя и хранилища закрытого ключа * в примере получает список сертификатов из всех доступных источников * build с последними изменениями * linter fixes
69 lines
1.9 KiB
TypeScript
Executable File
69 lines
1.9 KiB
TypeScript
Executable File
import 'cadesplugin';
|
|
import { rawCertificates, parsedCertificates } from '../__mocks__/certificates';
|
|
import { getContainerCertificates } from './getContainerCertificates';
|
|
|
|
const [rawCertificateMock] = rawCertificates;
|
|
const [parsedCertificateMock] = parsedCertificates;
|
|
|
|
const executionSteps = [
|
|
Symbol('step 0'),
|
|
Symbol('step 1'),
|
|
Symbol('step 2'),
|
|
Symbol('step 3'),
|
|
Symbol('step 4'),
|
|
Symbol('step 5'),
|
|
Symbol('step 6'),
|
|
Symbol('step 7'),
|
|
Symbol('step 8'),
|
|
Symbol('step 9'),
|
|
Symbol('step 10'),
|
|
];
|
|
|
|
const executionFlow = {
|
|
[executionSteps[0]]: {
|
|
Certificates: executionSteps[1],
|
|
Close: jest.fn(),
|
|
Open: jest.fn(),
|
|
},
|
|
[executionSteps[1]]: {
|
|
Find: jest.fn(() => executionSteps[2]),
|
|
},
|
|
[executionSteps[2]]: {
|
|
Find: jest.fn(() => executionSteps[3]),
|
|
},
|
|
[executionSteps[3]]: {
|
|
Count: executionSteps[4],
|
|
Item: jest.fn(() => executionSteps[5]),
|
|
},
|
|
[executionSteps[4]]: 1,
|
|
[executionSteps[5]]: {
|
|
IssuerName: executionSteps[8],
|
|
SubjectName: executionSteps[7],
|
|
Thumbprint: executionSteps[6],
|
|
ValidFromDate: executionSteps[9],
|
|
ValidToDate: executionSteps[10],
|
|
},
|
|
[executionSteps[8]]: rawCertificateMock.IssuerName,
|
|
[executionSteps[7]]: rawCertificateMock.SubjectName,
|
|
[executionSteps[6]]: rawCertificateMock.Thumbprint,
|
|
[executionSteps[9]]: rawCertificateMock.ValidFromDate,
|
|
[executionSteps[10]]: rawCertificateMock.ValidToDate,
|
|
};
|
|
|
|
window.cadesplugin.__defineExecutionFlow(executionFlow);
|
|
window.cadesplugin.CreateObjectAsync.mockImplementation(() => executionSteps[0]);
|
|
|
|
describe('getContainerCertificates', () => {
|
|
test('returns certificates list', async () => {
|
|
const certificates = await getContainerCertificates();
|
|
|
|
expect(certificates.length).toBeGreaterThan(0);
|
|
});
|
|
|
|
test('returns certificates with correct fields', async () => {
|
|
const [certificate] = await getContainerCertificates();
|
|
|
|
expect(certificate).toMatchObject(parsedCertificateMock);
|
|
});
|
|
});
|